일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 로또 회차
- 웹접근성
- IP차단
- 텍스트조절
- 바닐라 자바스크립트
- 기초
- 바닐라스크립트
- sqld52회차
- 애니메이션
- github
- 웹개발키워드
- SQL
- sqld
- 바닐라자바스크립트
- git
- CSS
- 코딩공부
- Python
- JSP
- TweenMax.js
- SQLD후기
- 마우스커서
- jQuery
- JS
- 웹표준
- VANILLA
- asp
- 팝업레이어
- 프론트앤드키워드
- Slide
- Today
- Total
단비의 코딩 공부 blog
[Python] study 2주차 본문
Python 구문 실행
파이썬 구문은 명령줄에 직접 작성하여 실행
print("Hello, World!")
출력 결과 :
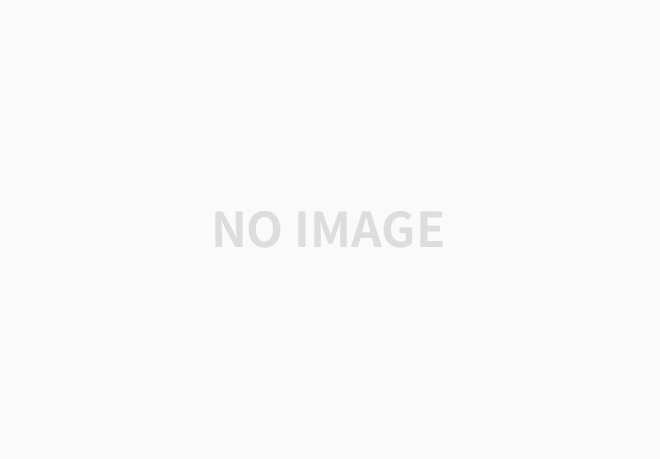
Python 들여쓰기
들여쓰기는 코드 줄 시작 부분의 공백을 나타내며, 다른 언어에서보다 파이썬에서의 들여쓰기는 매우 중요하다.
파이썬은 들여쓰기를 사용하여 블록을 나타낸다.
if 5 > 2:
print("Five is greater than two!")
출력 결과 :
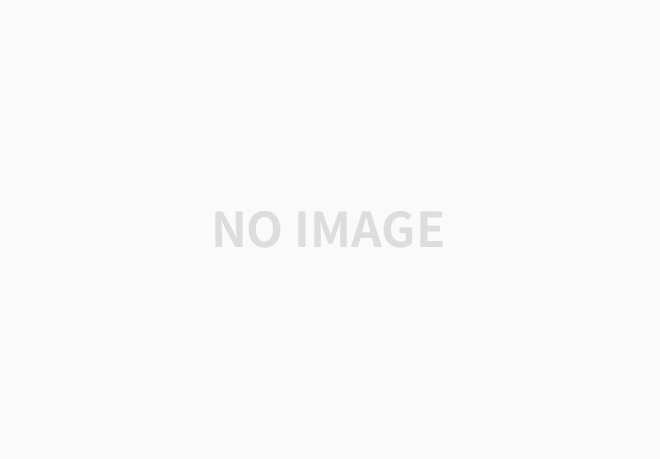
들여쓰기를 하지 않을 경우 오류가 발생한다.
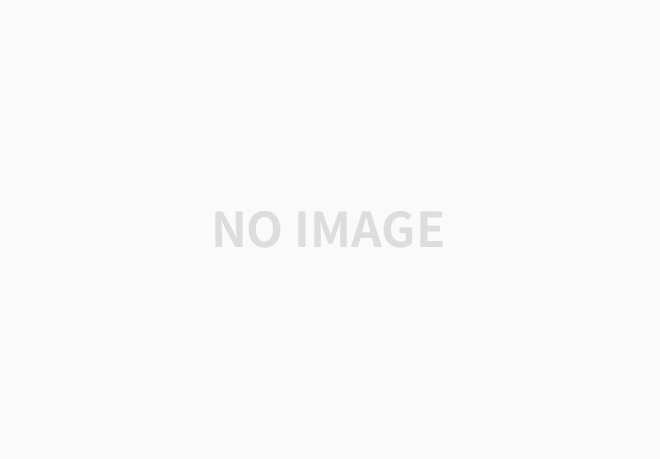
Python 주석
주석은 #로 시작하며, 파이썬은 이를 무시한다. 주석은 파이썬이 코드를 실행하는것을 방지하는 데에도 사용할 수 있다.
#한줄 주석
#This is a comment
print("Hello, World!")
print("Hello, World!") #This is a comment
#print("Hello, World!")
print("Cheers, Mate!")
여러줄 주석에도 #을 사용할 수 있고, 여러줄 문자열을 사용할 수도 있다.
파이썬은 변수에 할당되지 않은 문자열 리터럴을 무시하므로 코드에 여러 줄 문자열(""" - 삼중따옴표)을 추가하고 그 안에 주석을 넣을 수 있다.
#여러줄 주석
#This is a comment
#written in
#more than just one line
print("Hello, World!")
"""
This is a comment
written in
more than just one line
"""
print("Hello, World!")
출력 결과:
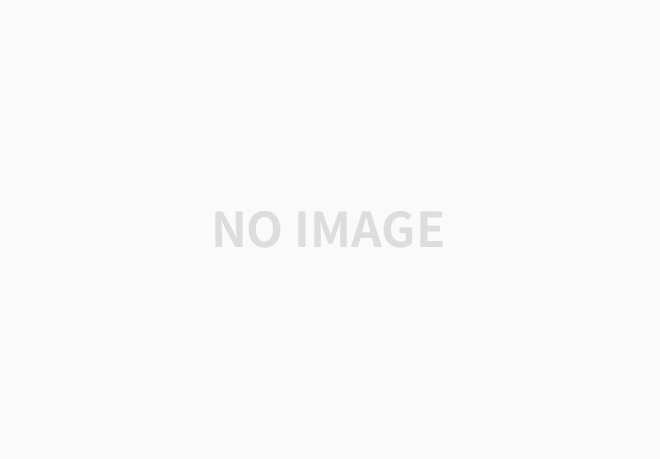
Python 변수
파이썬에서는 변수를 선언하는 명령이 없고, 처음 값을 할당하는 순간 생성된다.
x = 5
y = "John"
print(x)
print(y)
출력 결과:
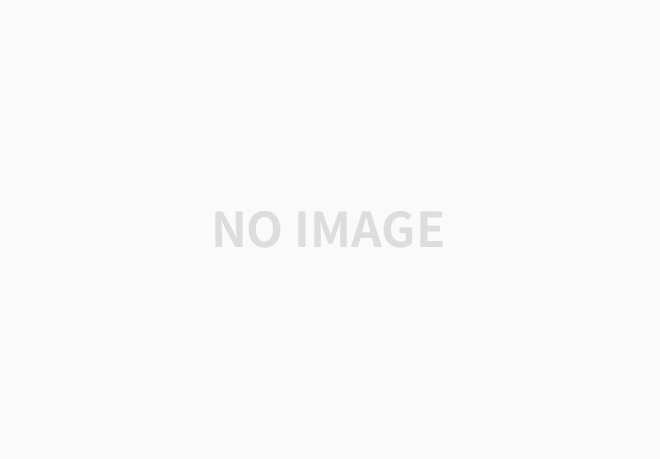
변수는 특정 유형으로 선언할 필요가 없으며, 설정된 후에 유형을 변경할 수도 있다.
x = 4 # x is of type int
x = "Sally" # x is now of type str
print(x)
출력 결과 :

변수의 데이터 유형을 지정하려면 캐스팅을 사용하면 된다.
x = str(3)
y = int(3)
z = float(3)
print(x)
print(y)
print(z)
출력 결과 :

함수를 사용하여 변수의 데이터 유형을 알 수 있다.
x = 5
y = "John"
print(type(x))
print(type(y))
출력 결과 :
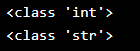
문자열 변수는 작은 따옴표나 큰따옴표를 사용하여 선언할 수 있다.
x = "John"
# is the same as
x = 'John'
출력 결과 :

변수 이름은 대소문자를 구분한다.
a = 4
A = "Sally"
#A will not overwrite a
출력 결과 :

Python 변수 - 이름
- 변수 이름은 문자나 밑줄 문자로 시작해야 합니다.
- 변수 이름은 숫자로 시작할 수 없습니다.
- 변수 이름에는 영숫자와 밑줄(Az, 0-9 및 _)만 포함할 수 있습니다.
- 변수 이름은 대소문자를 구분합니다(age, Age 및 AGE는 세 가지 다른 변수입니다).
- 변수 이름은 Python 키워드 중 하나일 수 없습니다 .
myvar = "John"
my_var = "John"
_my_var = "John"
myVar = "John"
MYVAR = "John"
myvar2 = "John"
잘못된 변수 이름 - > 오류
2myvar = "John"
my-var = "John"
my var = "John"
출력 결과 :

다중 단어 변수에는
- camel case - 첫 번째 단어를 제외한 각 단어는 대문자로 시작
myVariableName = "John"
- pascal case - 각 단어는 대문자로 시작
MyVariableName = "John"
- snake case
my_variable_name = "John"
Python 변수 - 할당
파이썬을 사용하여 한 줄로 여러 변수에 값을 할당할 수 있다.
x, y, z = "Orange", "Banana", "Cherry"
print(x)
print(y)
print(z)
출력 결과:
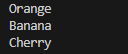
또, 한 줄에 여러 변수에 동일한 값을 할당할 수 있다.
x = y = z = "Orange"
print(x)
print(y)
print(z)
출력 결과:

목록, 튜플 등에 값 모음이 있는 경우 파이썬을 사용하면 값을 변수로 추출할 수 있다.
fruits = ["apple", "banana", "cherry"]
x, y, z = fruits
print(x)
print(y)
print(z)
출력 결과:
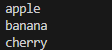
Python 변수 - 출력
파이썬 print() 함수는 변수를 출력하는데 사용 된다.
x = "Python is awesome"
print(x)
출력 결과:

함수에서는 여러 변수를 쉼표로 구분하여 출력한다.
x = "Python"
y = "is"
z = "awesome"
print(x, y, z)
출력 결과:

+연산자를 사용하여 여러변수를 출력할 수도 있다.
"Python "및 뒤에 있는 공백 문자를 주의해야한다. 공백 "is "문자가 없으면 결과는 "Pythonisawesome"이 된다.
x = "Python "
y = "is "
z = "awesome"
print(x + y + z)
출력 결과:

숫자의 경우 + 문자는 수학 연산자로 작동한다.
x = 5
y = 10
print(x + y)
출력 결과:

함수에서 문자열과 숫자를 연산자와 결합하면 오류가 난다.
x = 5
y = "John"
print(x + y)
출력 결과:

함수에 여러 변수를 출력하는 좋은 방법은 변수를 쉼표로 구분하는것이며, 이는 다양한 데이터 유형도 지원한다.
x = 5
y = "John"
print(x, y)
출력 결과:

Python 변수 - 전역 변수
함수 외부에서 생성된 변수를 전역 변수라고 한다. 전역변수는 함수 내부와 외부에서 사용할 수 있다.
x = "awesome"
def myfunc():
print("Python is " + x)
myfunc()
출력 결과:

함수 내에서 같은 이름의 변수를 생성하면 이 변수는 로컬변수가 되며 해당 함수 내에서만 사용할 수 있다.
동일한 이름의 전역 변수는 원래의 값과 함께 전역변수로 유지된다.
x = "awesome"
def myfunc():
x = "fantastic"
print("Python is " + x)
myfunc()
print("Python is " + x)
출력 결과:
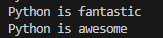
일반적으로 함수 내에서 변수를 생성하면 해당 변수는 로컬 변수이며 해당 함수 내에서만 사용할 수 있다.
함수 내에서 전역 변수를 생성할때 global 키워드를 사용할 수 있다.
def myfunc():
global x
x = "fantastic"
myfunc()
print("Python is " + x)
출력 결과:

또한 함수 내에서 전역 변수를 변경하려면 global 키워드를 사용한다.
x = "awesome"
def myfunc():
global x
x = "fantastic"
myfunc()
print("Python is " + x)
출력 결과:

Python 데이터유형
- 내장 데이터 유형
텍스트 유형: | str |
숫자 유형: | int, float, complex |
시퀀스 유형: | list, tuple, range |
매핑 유형: | dict |
세트 유형: | set,frozenset |
부울 유형: | bool |
바이너리 유형: | bytes, bytearray, memoryview |
없음 유형: | NoneType |
type()함수를 사용하여 모든 객체의 데이터 유형을 알 수 있다.
x = 5
print(type(x))
출력 결과:

Python 숫자
파이썬에는 세가지 숫자 유형이 있으며, 숫자 유형의 변수는 값을 할당하면 생성된다.
- int
- float
- complex
x = 1 # int
y = 2.8 # float
z = 1j # complex
print(type(x))
print(type(y))
print(type(z))
출력 결과:
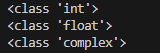
int 또는 정수 - 양수 또는 음수이며 소수점 이하 자릿수가 없고 길이가 무제한인 정수이다.
x = 1
y = 35656222554887711
z = -3255522
print(type(x))
print(type(y))
print(type(z))
출력 결과:
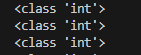
float - 하나 이상의 소수를 포함하는 양수 또는 음수 숫자이다.
x = 1.10
y = 1.0
z = -35.59
print(type(x))
print(type(y))
print(type(z))
출력 결과:
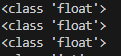
float은 10의 거듭제곱을 나타내는 "e"가 포함된 숫자일 수도 있다.
x = 35e3
y = 12E4
z = -87.7e100
print(type(x))
print(type(y))
print(type(z))
출력 결과:
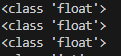
complex - 복소수는 허수부로 "j"를 사용한다.
x = 3+5j
y = 5j
z = -5j
print(type(x))
print(type(y))
print(type(z))
출력 결과:
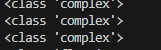
int(), float()및 complex() 메소드를 사용하여 한 유형에서 다른 유형으로 변환할 수 있다.
x = 1 # int
y = 2.8 # float
z = 1j # complex
#convert from int to float:
a = float(x)
#convert from float to int:
b = int(y)
#convert from int to complex:
c = complex(x)
print(a)
print(b)
print(c)
print(type(a))
print(type(b))
print(type(c))
출력 결과:
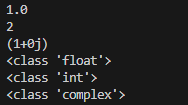
파이썬에는 난수를 만드는데 사용할수 있는 random() 내장 모듈이 있다.
import random
print(random.randrange(1, 10))
출력 결과 : 랜덤

Python 캐스팅
변수에 유형을 저장하려는 경우, 캐스팅 함수를 통해 가능하다.
파이썬은 객체지향 언어이기때문에 클래스를 사용하여 기본 유형을 포함한 데이터 유형을 정의한다.
- int() - 정수 리터럴, 부동 소수점 리터럴(모든 소수를 제거하여) 또는 문자열 리터럴(문자열이 정수를 나타내는 경우)에서 정수를 생성합니다.
- float() -정수 리터럴, 부동 소수점 리터럴 또는 문자열 리터럴에서 부동 소수점 숫자를 구성합니다(문자열이 부동 소수점 또는 정수를 나타내는 경우).
- str() - 문자열, 정수 리터럴, 부동 소수점 리터럴을 포함한 다양한 데이터 유형에서 문자열을 구성합니다.
x = int(1)
y = int(2.8)
z = int("3")
print(x)
print(y)
print(z)
x = float(1)
y = float(2.8)
z = float("3")
w = float("4.2")
print(x)
print(y)
print(z)
print(w)
x = str("s1")
y = str(2)
z = str(3.0)
print(x)
print(y)
print(z)
출력 결과 :
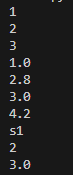
* 객체지향 언어 : 프로그램을 다수의 객체로 만들고, 이들끼리 서로 상호작용하도록 만드는 프로그래밍 언어
Python 문자열
파이썬의 문자열은 작은따옴표나 큰따옴표로 묶는다.
print("Hello")
print('Hello')
출력 결과:

변수에 문자열을 할당하는 것은 변수 이름 뒤에 등호와 문자열이 오는 방식으로 수행된다.
a = "Hello"
print(a)
출력 결과:

세 개의 따옴표(큰, 작은 상관X)를 사용하여 여러 줄 문자열을 변수에 할당할 수 있다.
a = """Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua."""
print(a)
a = '''Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua.'''
print(a)
출력 결과:
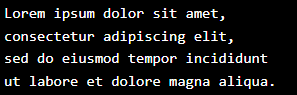
파이썬에는 문자 데이터 유형이 없으며 단일 문자는 단순히 길이가 1인 문자열이다.
대괄호를 사용하여 문자열 요소에 엑세스 할 수 있다. (0부터 시작)
a = "Hello, World!"
print(a[1])
출력 결과:

문자열은 배열이므로 for루프를 사용하여 문자열의 문자를 반복할 수 있다.
for x in "banana":
print(x)
출력 결과:
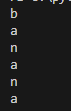
문자열의 길이를 얻기위해서는 len() 함수를 사용한다.
a = "Hello, World!"
print(len(a))
출력 결과:

문자열에 특정 문구나 문자가 있는지 확인하기 위해서는 in 키워드를 사용한다.
txt = "The best things in life are free!"
print("free" in txt)
txt = "The best things in life are free!"
if "free" in txt:
print("Yes, 'free' is present.")
출력 결과:

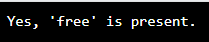
특정 문구나 문자가 문자열에 없는지 확인하려면 not in 키워드를 사용한다.
txt = "The best things in life are free!"
print("expensive" not in txt)
txt = "The best things in life are free!"
if "expensive" not in txt:
print("No, 'expensive' is NOT present.")
출력 결과:


Python 문자열 - Slicing
slicing 구문을 사용하여 다양한 문자를 반환할 수 있다. 물자열의 일부를 반환하려면 시작 인덱스와 끝 인덱스를 콜론으로 구분하여 지정해준다.
b = "Hello, World!"
print(b[2:5])
출력 결과:

시작인덱스를 생략하면 범위는 첫번째 문자에서 시작된다.
b = "Hello, World!"
print(b[:5])
출력 결과:

끝 인덱스를 생략하면 범위가 끝으로 이동한다.
b = "Hello, World!"
print(b[2:])
출력 결과:
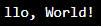
문자열 끝에서 시작하려면 음수 인덱스를 사용한다.
b = "Hello, World!"
print(b[-5:-2])
출력 결과:

Python 문자열 - 수정
upper() 메서드는 문자열을 대문자로 반환한다.
a = "Hello, World!"
print(a.upper())
출력 결과:
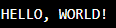
lower() 메서드는 문자열을 소문자로 반환한다.
a = "Hello, World!"
print(a.lower())
출력 결과:
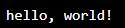
공백은 텍스트 앞/뒤에 있으며 제거할때는 strip() 메서드를 사용한다.
a = " Hello, World! "
print(a.strip()) # returns "Hello, World!"
출력 결과:
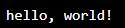
replace() 메서드는 문자열을 다른 문자열로 바꿔준다.
a = "Hello, World!"
print(a.replace("H", "J"))
출력 결과:
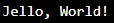
split() 메서드는 지정된 구분 기호 사이의 텍스트가 목록 항목이 되는 목록을 반환한다.
a = "Hello, World!"
print(a.split(",")) # returns ['Hello', ' World!']
출력 결과:

Python 문자열 - 연결
두 문자열을 연결하거나 결합하려면 + 연산자를 사용할 수 있다. 사이에 공백을 추가하려면 + " " + 을 추가해준다.
a = "Hello"
b = "World"
c = a + b
print(c)
a = "Hello"
b = "World"
c = a + " " + b
print(c)
출력 결과:

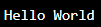
Python 문자열 - 형식
문자열과 숫자열은 결합하지 못한다.
age = 36
txt = "My name is John, I am " + age
print(txt)
출력 결과:
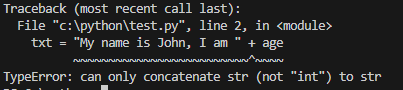
그러나 format() 함수를 메소드를 사용하면 결합할 수 있다.
format() 메서드는 전달된 인수를 가져와 형식을 지정하고 {}자리 표시자가 있는 문자열에 배치한다.
format() 메서드는 인수를 무제한으로 사용하며 각 자리 표시자에 배치된다.
age = 36
txt = "My name is John, and I am {}"
print(txt.format(age))
quantity = 3
itemno = 567
price = 49.95
myorder = "I want {} pieces of item {} for {} dollars."
print(myorder.format(quantity, itemno, price))
출력 결과:


인덱스 번호를 사용하여 {0} 인수가 올바른 자리 표시자에 배치되었는지 확인할 수 있다.
quantity = 3
itemno = 567
price = 49.95
myorder = "I want to pay {2} dollars for {0} pieces of item {1}."
print(myorder.format(quantity, itemno, price))
출력 결과:

Python 문자열 - 이스케이프 문자
문자열에 문자를 삽입하려면 이스케이프 문자를 사용한다. 이스케이프 문자는 백슬래시 \다음에 삽입하려는 문자이다.
txt = "We are the so-called \"Vikings\" from the north."
print(txt)
출력 결과:

Python 문자열 - 문자열 메서드
모든 문자열 메서드는 새로운 값을 반환하고 원래 문자열은 변경되지 않는다.
Method Description
capitalize() | Converts the first character to upper case |
casefold() | Converts string into lower case |
center() | Returns a centered string |
count() | Returns the number of times a specified value occurs in a string |
encode() | Returns an encoded version of the string |
endswith() | Returns true if the string ends with the specified value |
expandtabs() | Sets the tab size of the string |
find() | Searches the string for a specified value and returns the position of where it was found |
format() | Formats specified values in a string |
format_map() | Formats specified values in a string |
index() | Searches the string for a specified value and returns the position of where it was found |
isalnum() | Returns True if all characters in the string are alphanumeric |
isalpha() | Returns True if all characters in the string are in the alphabet |
isascii() | Returns True if all characters in the string are ascii characters |
isdecimal() | Returns True if all characters in the string are decimals |
isdigit() | Returns True if all characters in the string are digits |
isidentifier() | Returns True if the string is an identifier |
islower() | Returns True if all characters in the string are lower case |
isnumeric() | Returns True if all characters in the string are numeric |
isprintable() | Returns True if all characters in the string are printable |
isspace() | Returns True if all characters in the string are whitespaces |
istitle() | Returns True if the string follows the rules of a title |
isupper() | Returns True if all characters in the string are upper case |
join() | Joins the elements of an iterable to the end of the string |
ljust() | Returns a left justified version of the string |
lower() | Converts a string into lower case |
lstrip() | Returns a left trim version of the string |
maketrans() | Returns a translation table to be used in translations |
partition() | Returns a tuple where the string is parted into three parts |
replace() | Returns a string where a specified value is replaced with a specified value |
rfind() | Searches the string for a specified value and returns the last position of where it was found |
rindex() | Searches the string for a specified value and returns the last position of where it was found |
rjust() | Returns a right justified version of the string |
rpartition() | Returns a tuple where the string is parted into three parts |
rsplit() | Splits the string at the specified separator, and returns a list |
rstrip() | Returns a right trim version of the string |
split() | Splits the string at the specified separator, and returns a list |
splitlines() | Splits the string at line breaks and returns a list |
startswith() | Returns true if the string starts with the specified value |
strip() | Returns a trimmed version of the string |
swapcase() | Swaps cases, lower case becomes upper case and vice versa |
title() | Converts the first character of each word to upper case |
translate() | Returns a translated string |
upper() | Converts a string into upper case |
zfill() | Fills the string with a specified number of 0 values at the beginning |
Python 문자열 - Booleans
파이썬 불리언은 논리 자료형이며, True / Flase를 반환한다.
print(10 > 9)
print(10 == 9)
print(10 < 9)
출력 결과:

if문에서 조건을 실행하면 파이썬은 True또는 False를 반환한다.
a = 200
b = 33
if b > a:
print("b is greater than a")
else:
print("b is not greater than a")
print(bool("Hello"))
print(bool(15))
x = "Hello"
y = 15
print(bool(x))
print(bool(y))
출력 결과:



- True
- True 거의 모든 값은 일종의 콘텐츠가 있는지 평가된다.
- True을 제외한 모든 숫자는 0이다.
- 빈 문자열을 제외한 모든 문자열은 True다 .
print(bool("abc"))
print(bool(123))
print(bool(["apple", "cherry", "banana"]))

Python에는 객체가 특정 데이터 유형인지 확인하는 데 사용할 수 있는 isinstance() 함수 와 같이 부울 값을 반환하는 많은 내장 함수가 있다.
x = 200
print(isinstance(x, int))
출력 결과:

Python 문자열 - 연산자
연산자는 변수와 값에 대한 연산을 수행하는데 사용된다. 연산자 종류는 7가지가 있다.
- 산술 연산자
- 할당 연산자
- 비교 연산자
- 논리 연산자
- ID 연산자
- 회원 운영자
- 비트 연산자
- 산술 연산자
숫자 값과 함께 사용되어 일반적인 수학 연산을 수행한다.
Operator Name Example
+ | Addition | x + y |
- | Subtraction | x - y |
* | Multiplication | x * y |
/ | Division | x / y |
% | Modulus | x % y |
** | Exponentiation | x ** y |
// | Floor division | x // y |
- 할당 연산자
변수에 값을 할당하는 데 사용한다.
Operator Example Same As
= | x = 5 | x = 5 |
+= | x += 3 | x = x + 3 |
-= | x -= 3 | x = x - 3 |
*= | x *= 3 | x = x * 3 |
/= | x /= 3 | x = x / 3 |
%= | x %= 3 | x = x % 3 |
//= | x //= 3 | x = x // 3 |
**= | x **= 3 | x = x ** 3 |
&= | x &= 3 | x = x & 3 |
|= | x |= 3 | x = x | 3 |
^= | x ^= 3 | x = x ^ 3 |
>>= | x >>= 3 | x = x >> 3 |
<<= | x <<= 3 | x = x << 3 |
- 비교연산자
두값을 비교하는데 사용된다.
Operator Name Example
== | Equal | x == y |
!= | Not equal | x != y |
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal to | x >= y |
<= | Less than or equal to | x <= y |
- 논리 연산자
조건문을 결합하는 데 사용된다.
Operator Description Example
and | Returns True if both statements are true | x < 5 and x < 10 |
or | Returns True if one of the statements is true | x < 5 or x < 4 |
not | Reverse the result, returns False if the result is true | not(x < 5 and x < 10) |
- ID 연산자
객체가 같은지 비교하는 것이 아니라 실제로 동일한 메모리 위치를 가진 동일한 객체인지 비교하는데 사용된다.
perator Description Example
is | Returns True if both variables are the same object | x is y |
is not | Returns True if both variables are not the same object | x is not y |
-멤버십 연산자
시퀀스가 객체에 표시되는지 테스트하는데 사용된다.
Operator Description Example
in | Returns True if a sequence with the specified value is present in the object | x in y |
not in | Returns True if a sequence with the specified value is not present in the object | x not in y |
-비트 연산자
(이전) 숫자를 비교하는 데 사용된다.
perator Name Description Example
& | AND | Sets each bit to 1 if both bits are 1 | x & y |
| | OR | Sets each bit to 1 if one of two bits is 1 | x | y |
^ | XOR | Sets each bit to 1 if only one of two bits is 1 | x ^ y |
~ | NOT | Inverts all the bits | ~x |
<< | Zero fill left shift | Shift left by pushing zeros in from the right and let the leftmost bits fall off | x << 2 |
>> | Signed right shift | Shift right by pushing copies of the leftmost bit in from the left, and let the rightmost bits fall off | x >> 2 |
Python 문자열 - 연산자 우선순위
맨 위에서부터 우선 순위가 가장 높은 순서대로 정렬
perator Description
() | Parentheses |
** | Exponentiation |
+x -x ~x | Unary plus, unary minus, and bitwise NOT |
* / // % | Multiplication, division, floor division, and modulus |
+ - | Addition and subtraction |
<< >> | Bitwise left and right shifts |
& | Bitwise AND |
^ | Bitwise XOR |
| | Bitwise OR |
== != > >= < <= is is not in not in | Comparisons, identity, and membership operators |
not | Logical NOT |
and | AND |
or | OR |
Python Lists
list 는 단일 변수에 여러 항목을 저장하는데 사용한다. 대괄호를 사용하여 생성된다.
thislist = ["apple", "banana", "cherry"]
print(thislist)
출력 결과:

list 항목은 순서가 지정되고 변경 가능하며 중복 값을 허용한다.
list가 생성된 후 목록의 항목을 변경, 추가 및 제거할 수 있다.
또, list는 색인화 되어있으므로 동일한 값을 가진 항목이 있을 수도 있다.
thislist = ["apple", "banana", "cherry", "apple", "cherry"]
print(thislist)
출력 결과:

list에 포함된 항목 수를 확인하기위해서는 len()함수를 사용한다.
thislist = ["apple", "banana", "cherry"]
print(len(thislist))
출력 결과:

list 항목은 모든 데이터 유형이 될 수 있고 다양한 데이터 유형이 포함될 수 있다.
list1 = ["apple", "banana", "cherry"]
list2 = [1, 5, 7, 9, 3]
list3 = [True, False, False]
list1 = ["abc", 34, True, 40, "male"]
새로운 list를 만들때 list()생성자를 사용하는것도 가능하다.
thislist = list(("apple", "banana", "cherry")) # note the double round-brackets
print(thislist)
출력 결과:

Python Lists - 엑세스 항목
색은 번호를 참조하여 해당 항목에 엑세스 할 수 있다. (첫번째 항목의 인덱스는 0이다.)
thislist = ["apple", "banana", "cherry"]
print(thislist[1])
결과 출력:

음수 인덱스는 끝(뒤)부터 시작한다는 뜻이다.
thislist = ["apple", "banana", "cherry"]
print(thislist[-1])
결과 출력:

범위의 시작 위치와 끝 위치를 지정하여 인덱스 범위를 지정할 수 있다.
범위를 지정하면 반환 값은 지정된 항목이 포함된 새 list가 된다.
thislist = ["apple", "banana", "cherry", "orange", "kiwi", "melon", "mango"]
print(thislist[2:5])
출력 결과: * 검색은 인덱스 2(포함)에서 시작하여 인덱스 5(포함되지 않음)에서 끝난다.

목록 끝에서부터 검색을 시작하려면 음수로 인덱스를 지정해야한다.
thislist = ["apple", "banana", "cherry", "orange", "kiwi", "melon", "mango"]
print(thislist[-4:-1])
출력 결과:

항목이 존재하는지 확인할때에는 in 키워드를 사용한다.
thislist = ["apple", "banana", "cherry"]
if "apple" in thislist:
print("Yes, 'apple' is in the fruits list")
출력 결과:

Python Lists - List 항목 변경
특정 항목의 값을 변경하려면 색인 번호를 참조한다.
thislist = ["apple", "banana", "cherry"]
thislist[1] = "blackcurrant"
print(thislist)
출력 결과:

특정 범위 내의 항목 값을 변경하려면 새 값응로 목록을 정의하고 새 값을 삽입한다.
thislist = ["apple", "banana", "cherry", "orange", "kiwi", "mango"]
thislist[1:3] = ["blackcurrant", "watermelon"]
print(thislist)
출력 결과:

바꾸는 항목보다 더 많은 항목을 삽입하면 지정한 위치에 새 항목이 삽입되고 나머지 항목도 그에 따라 이동된다.
thislist = ["apple", "banana", "cherry"]
thislist[1:2] = ["blackcurrant", "watermelon"]
print(thislist)
출력 결과:

교체하는 것보다 적은 수의 항목을 삽입하면 지정한 위치에 새 항목이 삽입되고 나머지 항목은 그에 따라 이동된다.
thislist = ["apple", "banana", "cherry"]
thislist[1:3] = ["watermelon"]
print(thislist)
출력 결과:

기존 값을 바꾸지 않고 새 list 항목을 삽입하려면 inset() 메서드를 사용할 수 있다.
thislist = ["apple", "banana", "cherry"]
thislist.insert(2, "watermelon")
print(thislist)
출력 결과:

Python Lists - 항목 추가
list 끝에 항목을 추가 하려면 append() 메서드를 사용한다.
thislist = ["apple", "banana", "cherry"]
thislist.append("orange")
print(thislist)
출력 결과:

지정된 인덱스에 목록 항목을 삽입하려면 inset() 메서드를 사용한다.
thislist = ["apple", "banana", "cherry"]
thislist.insert(1, "orange")
print(thislist)
출력 결과:

다른 list의 요소를 현재 목록에 추가하려면 extend() 메서드를 사용한다. 요소는 몰록 끝에 추가된다
thislist = ["apple", "banana", "cherry"]
tropical = ["mango", "pineapple", "papaya"]
thislist.extend(tropical)
print(thislist)
출력 결과:

extent()메서드는 목록을 추가할 필요가 없으며, 반복 가능한 객체(튜플, 사전, 세트 등)를 추가할 수 있다.
thislist = ["apple", "banana", "cherry"]
thistuple = ("kiwi", "orange")
thislist.extend(thistuple)
print(thislist)
출력 결과:

Python Lists - 항목 제거
remove() 메서드는 지정된 항목을 제거한다.
thislist = ["apple", "banana", "cherry"]
thislist.remove("banana")
print(thislist)
출력 결과:

지정된 값을 가진 항목이 두 개 이상인 경우 remove() 메서드는 첫 번째 항목을 제거한다.
thislist = ["apple", "banana", "cherry", "banana", "kiwi"]
thislist.remove("banana")
print(thislist)
출력 결과:

pop() 메서드는 지정된 인덱스를 제거한다.
thislist = ["apple", "banana", "cherry"]
thislist.pop()
print(thislist)
출력 결과:

del키워드는 지정된 인덱스도 제거할 수 있다.
thislist = ["apple", "banana", "cherry"]
del thislist[0]
print(thislist)
thislist = ["apple", "banana", "cherry"]
del thislist
출력 결과:


clear() 메서드는 목록을 비울 때 사용한다. 목록은 남아있지만 내용은 남아있지 않다.
thislist = ["apple", "banana", "cherry"]
thislist.clear()
print(thislist)
출력 결과:

Python Lists - 루프
for 루프를 사용하여 list 항목을 반복할 수 있다.
thislist = ["apple", "banana", "cherry"]
for x in thislist:
print(x)
출력 결과:

색인 번호를 참조하여 목록 항목을 반복할 수도 있다. range() 및 len() 함수를 사용하여 반복 가능한 list를 만든다.
thislist = ["apple", "banana", "cherry"]
for i in range(len(thislist)):
print(thislist[i])
출력 결과:

while루프를 사용하여 목록 항목을 반복할 수 있다.
len() 함수를 사용하여 목록의 길이를 결정한 다음 0에서 시작하여 해당 인덱스를 참조하여list 항목을 반복한다.
각 반복 후 인덱스에 1씩 늘린다.
thislist = ["apple", "banana", "cherry"]
i = 0
while i < len(thislist):
print(thislist[i])
i = i + 1
#쉬운구문
thislist = ["apple", "banana", "cherry"]
[print(x) for x in thislist]
출력 결과:

Python Lists - 이해
for를 사용하여 "a"가 포함된 단어만 포함하는 새 목록을 만든다.
fruits = ["apple", "banana", "cherry", "kiwi", "mango"]
newlist = []
for x in fruits:
if "a" in x:
newlist.append(x)
print(newlist)
#쉬운구문
fruits = ["apple", "banana", "cherry", "kiwi", "mango"]
newlist = [x for x in fruits if "a" in x]
print(newlist)
출력 결과:

newlist = [expression for item in iterable if condition == True]
newlist = [x for x in fruits if x != "apple"] #!부정
range() 함수를 사용하여 반복 개체를 만들 수 있다.
newlist = [x for x in range(10)]
print(newlist)
newlist = [x for x in range(10) if x < 5]
print(newlist)
출력 결과:


Python Lists - 정렬
list 개체에는 기본적으로 목록을 영숫자순, 오름차순으로 정렬하는 sort() 메서드가 있다.
대문자가 소문자보다 먼저 정렬된다.
thislist = ["orange", "mango", "kiwi", "pineapple", "banana"]
thislist.sort()
print(thislist)
thislist = [100, 50, 65, 82, 23]
thislist.sort()
print(thislist)
출력 결과:


내림차순으로 정렬하려면 reverse = True키워드를 사용한다.
thislist = ["orange", "mango", "kiwi", "pineapple", "banana"]
thislist.sort(reverse = True)
print(thislist)
thislist = [100, 50, 65, 82, 23]
thislist.sort(reverse = True)
print(thislist)
출력 결과:


key = function 을 사용하여 자신만의 함수를 정의할 수 있다. 가장 낮은 숫자부터 반환한다.
def myfunc(n):
return abs(n - 50)
thislist = [100, 50, 65, 82, 23]
thislist.sort(key = myfunc)
print(thislist)
출력 결과:

대소문자를 구분하지 않는 정렬 기능을 원하면 str.lower을 사용한다.
thislist = ["banana", "Orange", "Kiwi", "cherry"]
thislist.sort(key = str.lower)
print(thislist)
출력 결과:

역순을 출력하고 싶을땐 reserve() 메서드를 사용한다.
thislist = ["banana", "Orange", "Kiwi", "cherry"]
thislist.reverse()
print(thislist)
출력 결과:

Python Lists - 복사
copy() 메서드 / list() 메서드를 사용하며면 복사본을 만들 수 있다.
thislist = ["apple", "banana", "cherry"]
mylist = thislist.copy()
print(mylist)
thislist = ["apple", "banana", "cherry"]
mylist = list(thislist)
print(mylist)
출력 결과:

Python Lists - 조인
두 개 이상의 목록을 결합하거나 연결하는 방법에는 여러가지 방법이 있다. +, for x in, extend()
list1 = ["a", "b", "c"]
list2 = [1, 2, 3]
list3 = list1 + list2
print(list3)
list1 = ["a", "b" , "c"]
list2 = [1, 2, 3]
for x in list2:
list1.append(x)
print(list1)
list1 = ["a", "b" , "c"]
list2 = [1, 2, 3]
list1.extend(list2)
print(list1)
출력 결과:
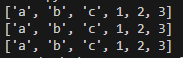
Python Lists - 메서드
Method Description
append() | Adds an element at the end of the list |
clear() | Removes all the elements from the list |
copy() | Returns a copy of the list |
count() | Returns the number of elements with the specified value |
extend() | Add the elements of a list (or any iterable), to the end of the current list |
index() | Returns the index of the first element with the specified value |
insert() | Adds an element at the specified position |
pop() | Removes the element at the specified position |
remove() | Removes the item with the specified value |
reverse() | Reverses the order of the list |
sort() | Sorts the list |
'Python' 카테고리의 다른 글
[Python] study 5주차 (1) | 2024.04.26 |
---|---|
[Python] study 4주차 (0) | 2024.04.17 |
[Python] study 3주차 (0) | 2024.04.12 |
[Python] study 1주차 (0) | 2024.04.04 |